Spring MVC vs Spring Boot: Which Framework to Choose?
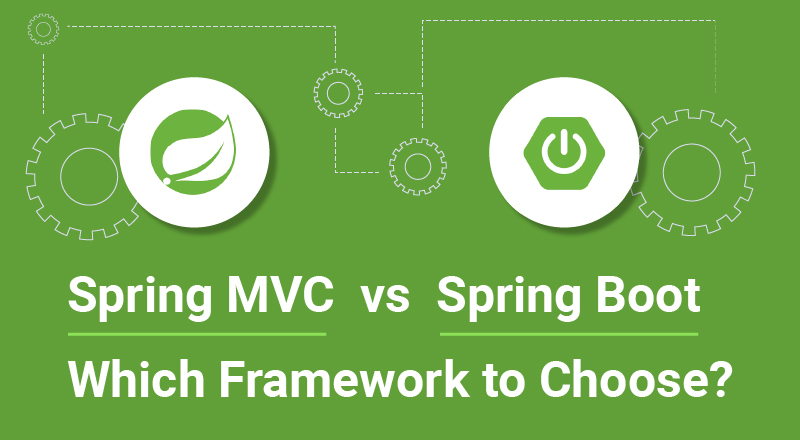
When it comes to building Java web applications, Spring Framework is a popular choice due to its robust features and flexibility. However, within the Spring ecosystem, you’ll often hear about two key components: Spring MVC and Spring Boot.
What is Spring MVC?
Spring MVC, which stands for “Spring Model-View-Controller,” is a framework within the broader Spring Framework that is specifically designed for building web applications.
It provides a structured approach to developing web applications based on the Model-View-Controller (MVC) architectural pattern. Spring MVC is a robust framework for building web applications that adhere to the MVC architectural pattern.
It simplifies the development of web-based systems by providing a structured approach to request handling, view rendering, and data processing. Developers often use Spring MVC for creating interactive and data-driven websites in Java-based applications.
What is Spring Boot?
Spring Boot is a popular open-source framework within the Spring ecosystem that simplifies the development, configuration, and deployment of Java applications. It’s designed to accelerate the process of building production-ready applications with minimal effort and boilerplate code.
Spring Boot simplifies Java application development by providing a set of defaults, embedded servers, and a streamlined development process. It’s an excellent choice for developers who want to build robust, production-ready applications quickly while maintaining the flexibility to customize configurations as needed.
Spring Web MVC’s Robust Features
Spring Web MVC framework stands as a beacon of efficiency in the web development landscape, offering a clear separation of roles that ensures a modular and organized approach.
Each role, from the controller to the view resolver, is designed to be fulfilled by a specialized object, streamlining the development process.
The framework’s power lies in its configuration capabilities, allowing both framework and application classes to be managed as JavaBeans. This feature facilitates effortless referencing across different contexts, enhancing collaboration between web controllers, business objects, and validators.
Spring Web MVC’s hallmark is its adaptability and flexibility. It empowers developers to define controller method signatures tailored to specific needs, utilizing annotations like @RequestParam, @RequestHeader, and @PathVariable to cater to diverse scenarios.
A key advantage of Spring Web MVC is the reuse of business code, eliminating the redundancy often seen in other frameworks. Developers can utilize existing business objects as command or form objects, avoiding the need to extend framework-specific base classes.
Customizable binding and validation mechanisms are another standout feature. Spring Web MVC handles type mismatches as application-level validation errors, retaining the offending values and facilitating localized date and number binding.
The framework’s handler mapping and view resolution are highly customizable, offering a range of strategies from simple URL-based configurations to complex resolution techniques, providing more flexibility than traditional web MVC frameworks.
Flexible model transfer is made possible through a name/value Map, ensuring seamless integration with various view technologies. Additionally, Spring Web MVC offers customizable locale and theme resolution, comprehensive support for JSPs, and a robust tag library that simplifies data binding and theming.
Introduced in Spring 2.0, the JSP form tag library greatly simplifies form creation in JSP pages. Moreover, Spring Web MVC utilizes WebApplicationContext containers to manage beans with lifecycles scoped to the current HTTP request or session, further enhancing its functionality.
By harnessing these features, Spring Web MVC provides developers with a powerful toolkit to create dynamic and responsive web applications.
Feature References by: Spring.io
Features of Spring Boot
- Opinionated Defaults: Spring Boot provides sensible default configurations and settings for various aspects of application development. This “opinionated” approach reduces the need for extensive manual configuration, allowing developers to get started quickly.
- Embedded Servers: Spring Boot includes embedded servlet containers like Tomcat, Jetty, and Undertow, eliminating the need for manual server setup and configuration.
- Standalone Applications: Spring Boot applications are self-contained and can be run as standalone JAR files. They are packaged with embedded servers, simplifying deployment and scaling.
- Externalized Configuration: Spring Boot supports externalized configuration through properties files or YAML files. Developers can configure applications for different environments (development, production, etc.) without code changes.
- Microservices and Cloud-Native Support: Spring Boot is well-suited for building microservices and cloud-native applications. It provides features like service discovery, load balancing, and externalized configuration for microservices architectures.
Spring MVC Benefits
- Modularity: Spring MVC encourages the separation of concerns through the MVC architectural pattern. This modularity makes it easier to develop, test, and maintain different parts of your application independently.
- Flexibility: Spring MVC provides flexibility in choosing the view technology (e.g., JSP, Thymeleaf, FreeMarker) and configuring the application using XML or Java-based configuration.
- View Resolution: Developers can choose from various view technologies to render web pages, making it adaptable to different project requirements.
- Interceptors: Interceptors allow you to perform pre-processing and post-processing tasks on requests and responses, such as authentication, logging, or data transformation.
Spring Boot Benefits
- Rapid Development: Spring Boot provides opinionated defaults and auto-configuration, reducing the need for manual setup. Developers can start coding quickly without getting bogged down by boilerplate configuration.
- Standalone Applications: Spring Boot applications are self-contained and can be run as standalone JAR files. They include embedded servers, simplifying deployment, and scaling.
- Testing Support: Spring Boot provides utilities and annotations for testing, simplifying the writing of unit tests and integration tests for your application.
- Dependency Management: It simplifies dependency management through predefined “starters” for common tasks and technologies. This reduces the effort required to include libraries and tools in your project.
Limitations of Spring MVC
- Configuration Complexity: Configuring Spring MVC can be complex, especially for newcomers. It often involves XML or Java-based configuration, which can lead to steep learning curves.
- Boilerplate Code: Spring MVC applications can sometimes involve writing a significant amount of boilerplate code, particularly when dealing with XML configurations and controller mappings.
- Limited Support for Reactive Programming: Spring MVC is designed primarily for traditional servlet-based applications. If you need to build fully reactive applications, you may need to consider other Spring projects like Spring WebFlux.
- Performance Overheads: While Spring MVC offers excellent features and extensibility, these can come with performance overheads, especially if not configured optimally. In some cases, this might not be suitable for high-performance, low-latency applications.
Limitations of Spring Boot
- Opinionated Defaults: While opinionated defaults can accelerate development, they might not align with specific project requirements. Developers may need to override these defaults, which can be challenging.
- Overhead: Spring Boot’s auto-configuration and embedded servers can introduce some performance overhead. For certain high-performance or low-latency applications, this overhead may be a concern.
- Potential for Magic: Spring Boot’s magic (automatic configuration) can sometimes lead to situations where developers are unsure of how certain features are enabled, making debugging, and troubleshooting more challenging.
- Limited to Java Applications: Spring Boot is primarily designed for Java applications. While it can be used with other JVM languages, it may not be the best choice for applications developed in languages outside the JVM ecosystem.
Read: Securing Java Applications Guide
Spring MVC vs. Spring Boot: Key Similarities
- Dependency on the Spring Framework: Both Spring MVC and Spring Boot are built on top of the Spring Framework.
- Java-Based: Both frameworks are Java-based and leverage the Java ecosystem, including libraries and tools.
- Component-Based: Spring MVC and Spring Boot frameworks encourage the use of components like controllers, services, and repositories
- Annotation-Driven: Both frameworks heavily use annotations for configuration.
- Externalized Configuration: Both frameworks support externalized configuration.
- IoC Container: Spring Boot and Spring MVC rely on the Inversion of Control (IoC) container provided by the Spring Framework
- Testing Support: Both Spring MVC and Spring Boot provide support for unit testing and integration testing.
Spring MVC vs Spring Boot: Key Differences to Know
Parameters |
Spring MVC |
Spring Boot |
Framework Type |
Spring MVC is a part of the broader Spring Framework, which provides a wide range of components for various purposes. |
Spring Boot is a framework built on top of the Spring Framework with a focus on rapid application development. |
Configuration |
Configuration is explicit, often requiring XML or Java-based configuration files. |
Embraces convention over configuration but allows for customizations through properties files or Java code. |
Project Setup |
Developers must set up the project structure and configure components manually. |
Easier to learn and suitable for both newcomers and experienced Spring developers. |
Server Configuration |
Manual configuration of the servlet container (e.g., Tomcat) is typically required |
Project setup is streamlined, thanks to opinionated defaults and a predefined project structure. |
Dependency Management |
Developers need to manage project dependencies explicitly. |
Includes embedded servlet containers, eliminating manual server configuration. |
Flexibility |
Spring MVC doesn’t prescribe any particular project structure, giving developers the freedom to organize their code as they see fit. |
Simplifies dependency management with Spring Boot Starter dependencies and auto-configuration. |
Learning Curve |
Due to its configurational nature, Spring MVC can have a steeper learning curve for newcomers to the Spring ecosystem. |
Ideal for projects with straightforward requirements but may have limitations for highly customized applications. |
Primary Feature |
The primary feature of the Spring Framework is dependency injection. |
The primary feature of Spring Boot is Autoconfiguration. It automatically configures the classes based on the requirement. |
Server |
For testing the Spring project, it’s necessary to explicitly configure the server. |
Spring Boot provides embedded server like Tomcat, Jetty, etc. |
Use Case |
It is well-suited for projects with complex configurations, specific customization requirements, or where fine-grained control over components is essential. |
It is ideal for rapid application development, prototyping, and projects with standard configurations. It is particularly useful for microservices and containerized applications. |
Spring MVC vs Spring Boot: The Conclusion
It’s important to note that many of these drawbacks can be addressed or mitigated with proper planning, understanding of the frameworks, and adherence to best practices.
Additionally, the choice between Spring MVC and Spring Boot should align with the specific project requirements, team expertise, and development goals. In some cases, combining both frameworks in a single project can also be a viable approach to leverage their respective strengths.
In conclusion, the choice between Spring MVC and Spring Boot depends on your project’s specific needs. Both frameworks have their strengths, and understanding your project’s requirements and your team’s expertise will guide you toward the right choice. Ultimately, Spring Boot’s simplicity and productivity gains have made it a popular choice for many modern Java web applications.
SPEC INDIA, as your single stop IT partner has been successfully implementing a bouquet of diverse solutions and services all over the globe, proving its mettle as an ISO 9001:2015 certified IT solutions organization. With efficient project management practices, international standards to comply, flexible engagement models and superior infrastructure, SPEC INDIA is a customer’s delight. Our skilled technical resources are apt at putting thoughts in a perspective by offering value-added reads for all.
Delivering Digital Outcomes To Accelerate Growth
Let’s Talk